Chapter 4:
Create your database
We’ll use Data Tables to store feedback from our users and then publish our app online for anyone to use.
Data Tables are an out-of-the-box option for data storage in Anvil, and they’re backed by PostgreSQL.
Step 1: Add a Data Table to store your feedback
In the sidebar menu on the left, click the database icon .
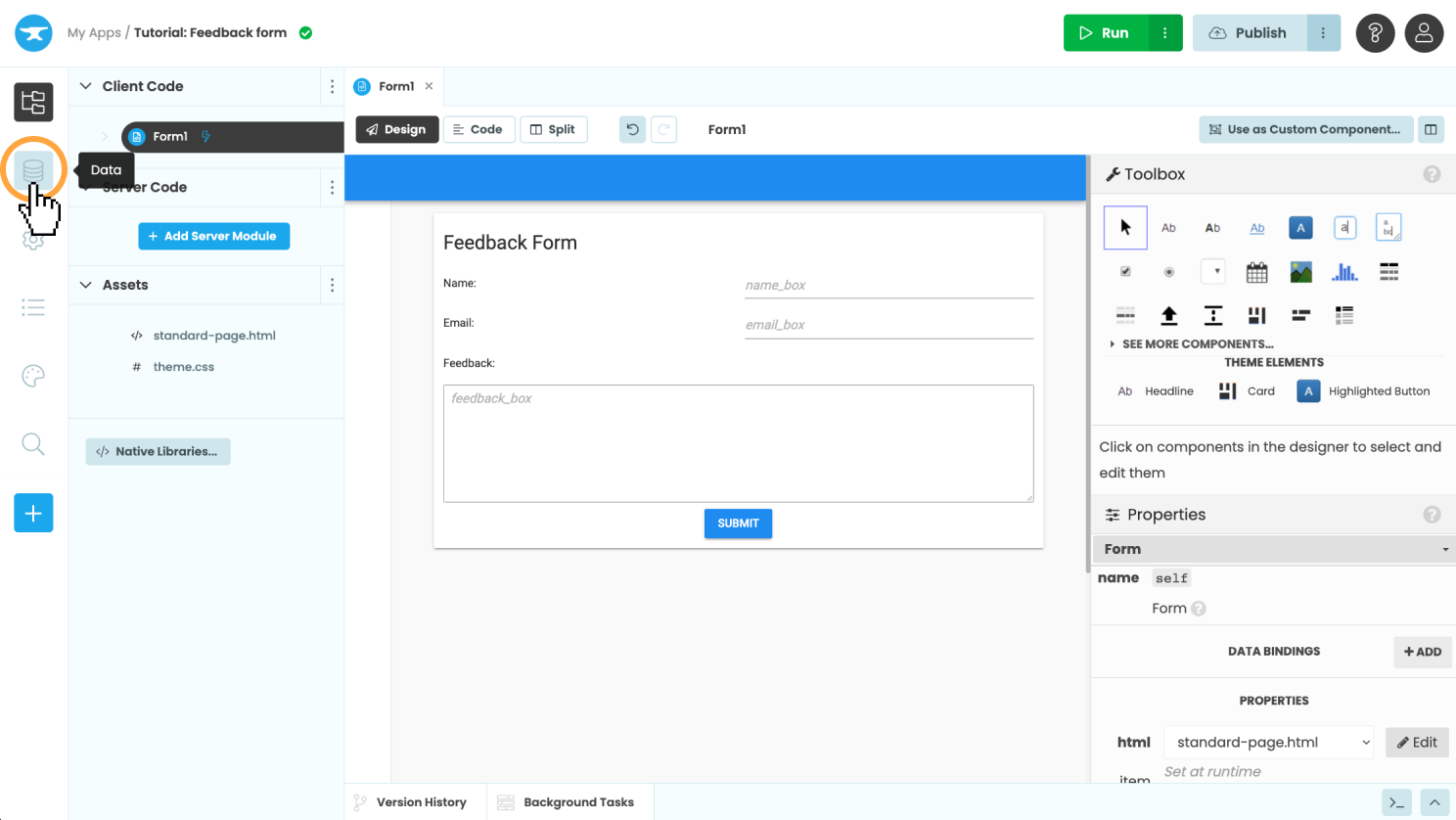
Click on ‘+ Add Table’ and name it ‘feedback’.
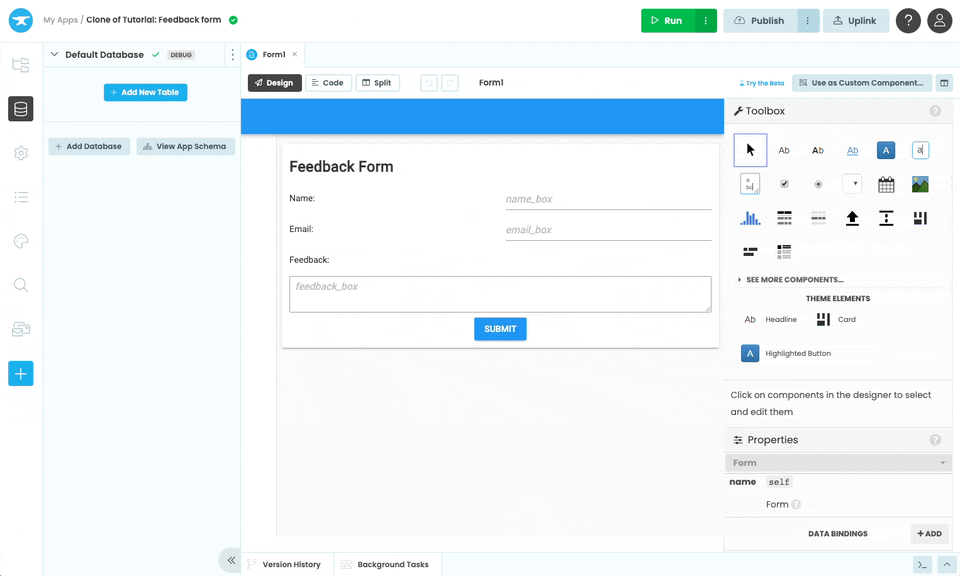
Renaming the table
Step 2: Set up your table
Now, let’s set up our “feedback” table with the following columns:
name
(Text column)email
(Text column)feedback
(Text column)created
(Date and Time column)
Here’s how to do that:
- Add a column for the name of the person submitting feedback, by clicking ‘+ New Column’. Call this column ’name’ and under Column Type select
Text
. Click ‘Ok’. - Keep adding columns until our Data Table has the structure we described above. Column titles are case sensitive, so let’s stick to lower case.
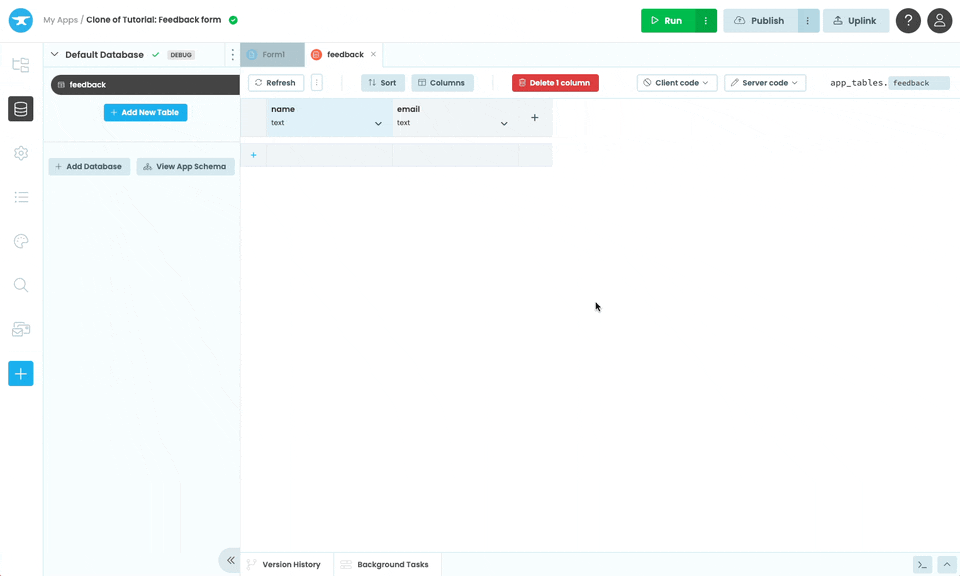
Our Data Table should look something like this:
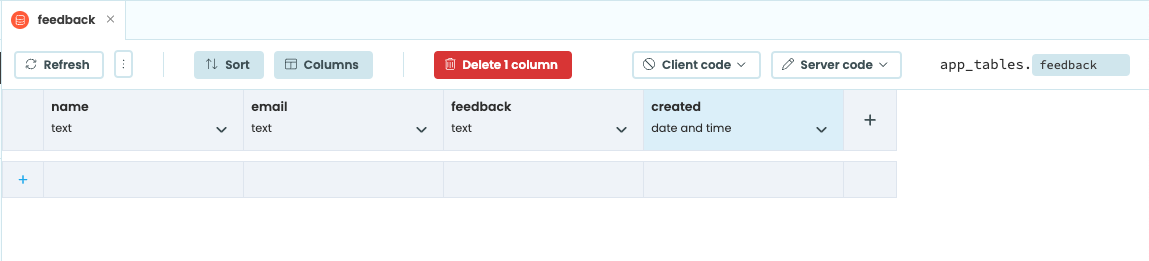
The finished table
Step 3: Update your server function
Now, let’s update our server function so it adds the feedback to our data table using Anvil’s app_tables
API.
Go to our Server Module, and modify the send_feedback
function to look like this.
@anvil.server.callable
def send_feedback(name, email, feedback):
# Send yourself an email each time feedback is submitted
anvil.email.send(#to="noreply@anvil.works", # Change this to your email address!
subject=f"Feedback from {name}",
text=f"""
A new person has filled out the feedback form!
Name: {name}
Email address: {email}
Feedback:
{feedback}
""")
app_tables.feedback.add_row(
name=name,
email=email,
feedback=feedback,
created=datetime.now()
)
Server Modules cannot be edited or seen by the user, so we can trust them to do what we tell them. This is why we’ll use a Server Module to write to the Data Table.
We’re using the datetime library to set the ‘created’ column to the current date and time, so you’ll also need to import the datetime
class at the top of your Server Module:
from datetime import datetime
To finish, let’s publish our app online.
Step 4: Publish your app
We can easily publish our app online for anyone to use. Click the ‘Publish’ button at the top right of the editor, then select ‘Publish this app’ and use the public URL provided or enter your own.
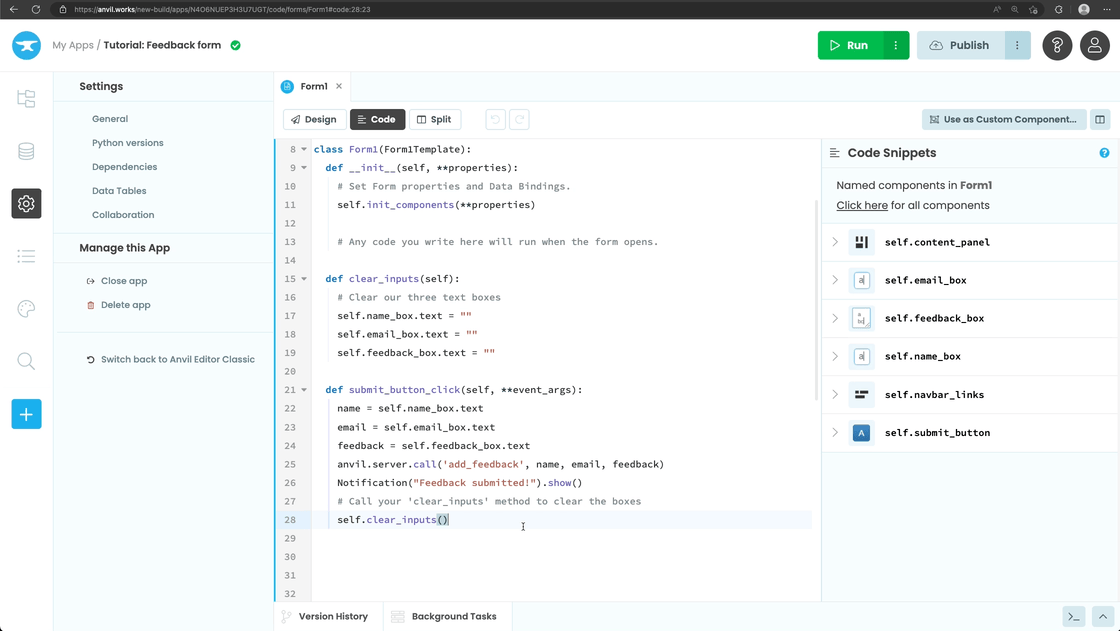
Publishing an app and changing its URL
Fill in a name, email address, and some feedback and click Submit.
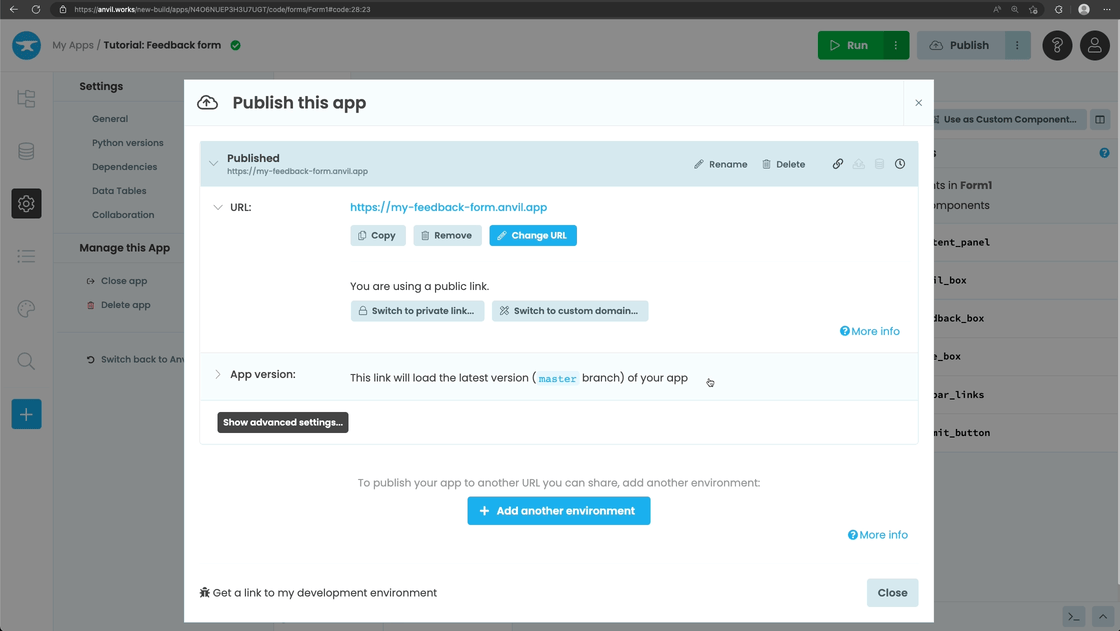
Using the deployed app
And that’s it, we’ll receive an email letting us know someone has filled out our feedback form and our app will store the feedback in our database!
And that’s it. You’ve just built a working database-backed feedback app in Anvil.
You can use the following link to clone the finished app and explore it yourself:
In the next section, you can challenge yourself to reinforce what you’ve learnt and learn more!
If you’d rather not try the challenge, check out the below section to see what’s next.
What next?
Head to the Anvil Learning Centre for more tutorials, or head to our examples page to see how to build some complex apps in Anvil.
Build Database-Backed Apps
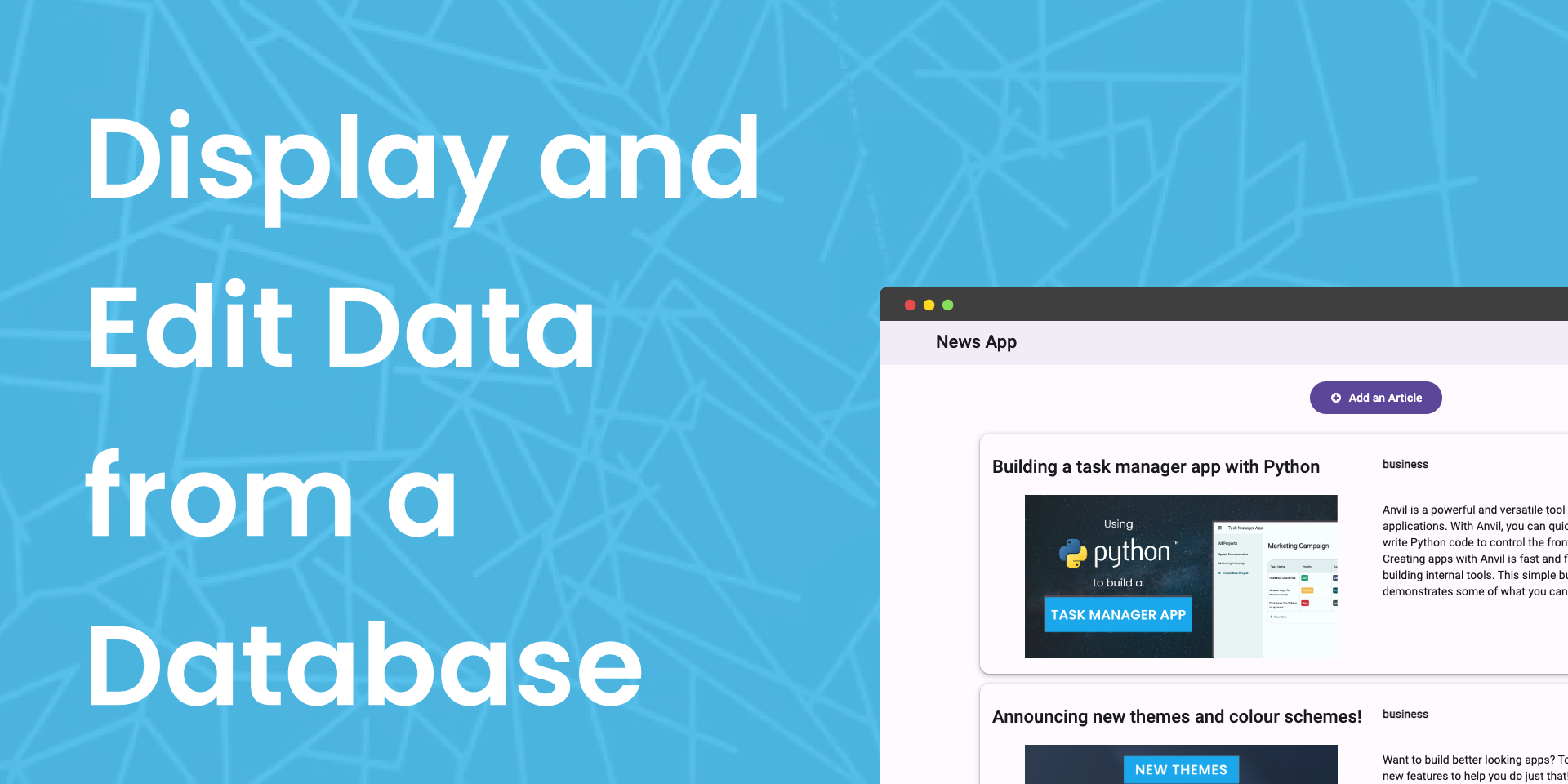
In this tutorial, you’ll learn how to do all of those things and more in Anvil.
Display data on a dashboard
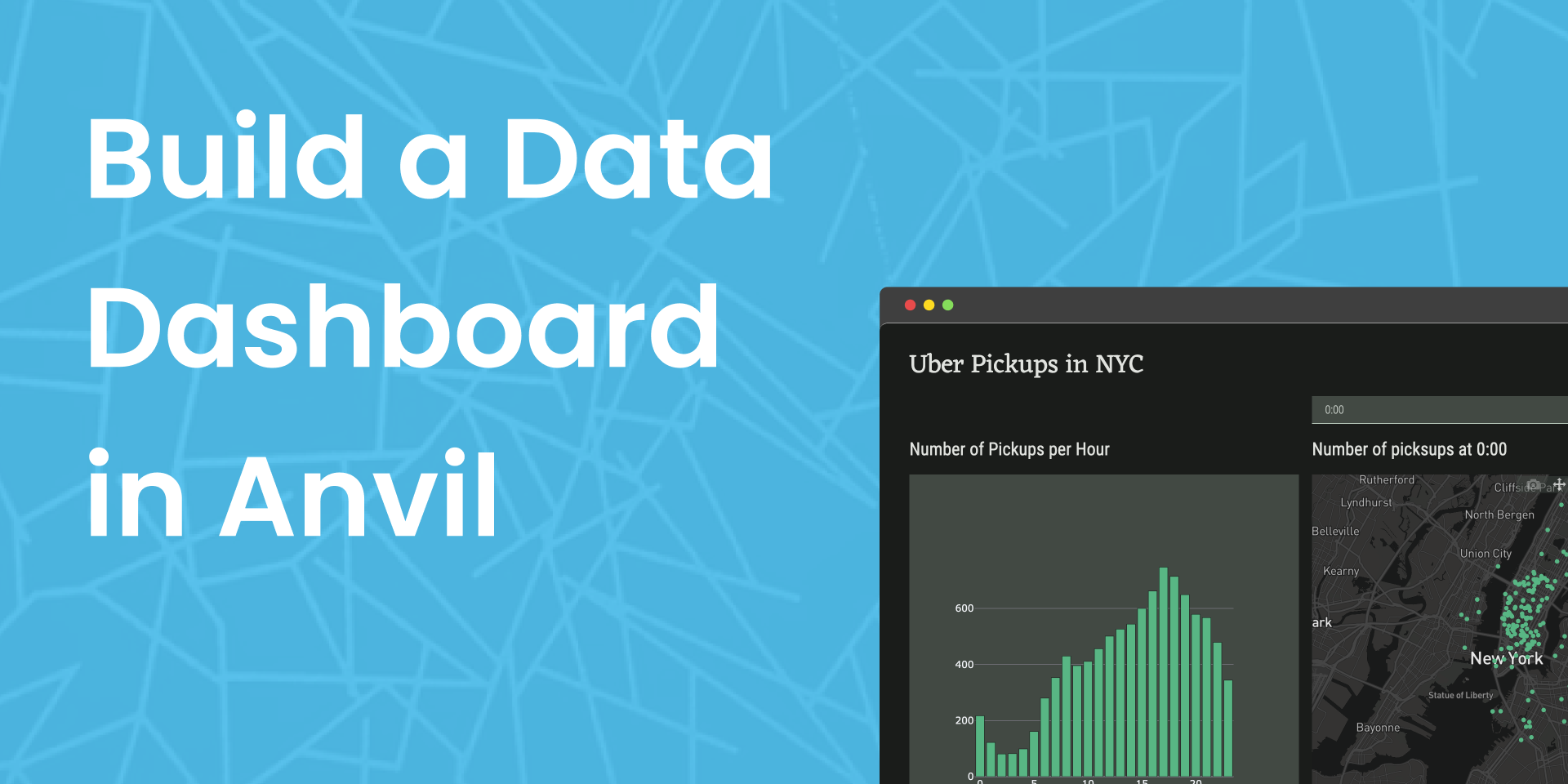
Deploy data science to the web with Deepnote
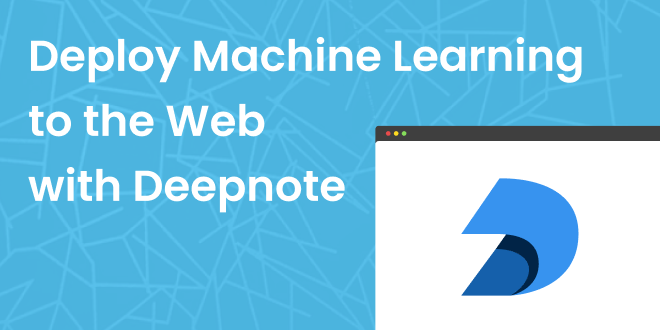
Generate PDF Invoices with Python
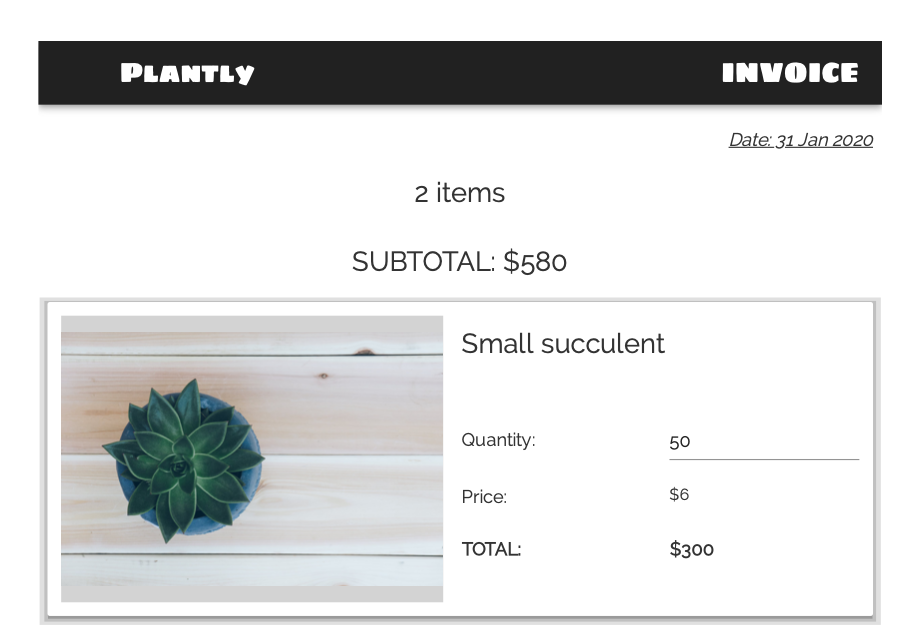
See a large-scale ticketing system, built with Anvil
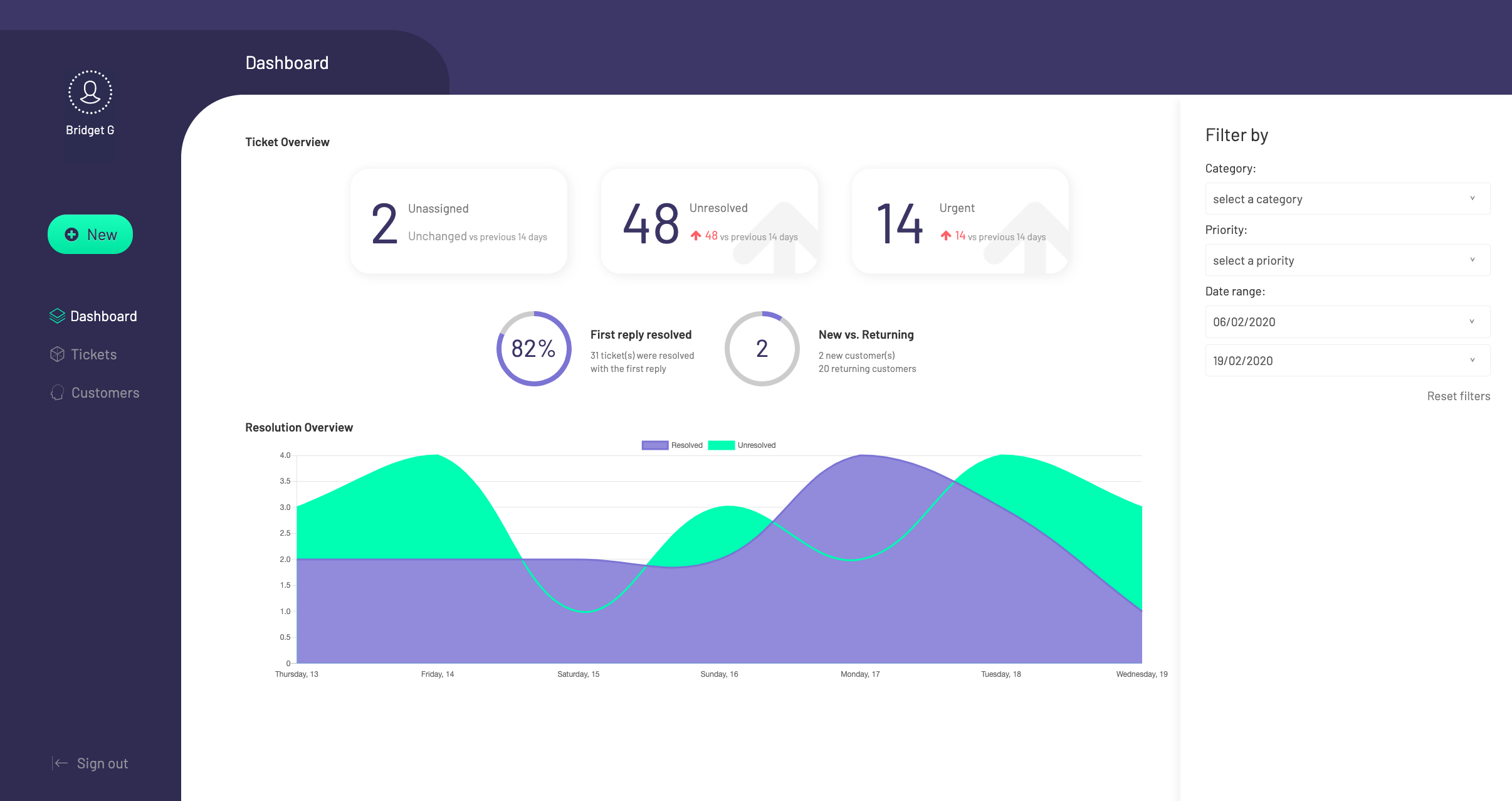