Author: Stuart Cork
This library contains a popover component, which allows you to add popover functionality to Links and Buttons:
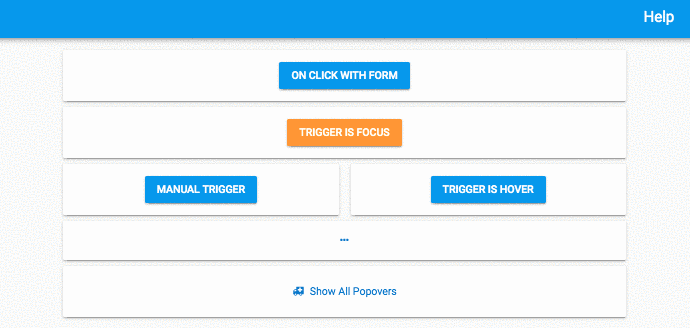
The popovers are highly configurable; play with the settings in the example app to explore.
This library is completely free to use, so click on the clone link and use it in your app!
When you clone the library, you will also get an example app that shows you how to add popover functionality to your app.
You can add it to an existing app using the App Dependencies dialog.
How it works
Two methods are added to the Button and Link classes:
def popover(self, content,
title='',
placement = 'right',
trigger = 'click',
animation = True,
delay={ "show": 100, "hide": 100 }
):
"""should be called by a button or link
content - either a string or an anvil component or Form
title - a string
placement - right, left, top, bottom (for left/right best to have links and buttons inside flow panels)
trigger - manual, focus, hover, click
animation - True or False
delay - {'show': 100, 'hide': 100}
if the content is a form then the form will inherit a self.popper attribute"""
if isinstance(content, str):
html = False
else:
html = True
# add the popper to the content form
content.popper = self
js.call_js('popover', self, content, title, placement, trigger, animation, delay, html)
def pop(self,behaviour):
""" trigger some behaviour of the popover.
behaviour can be any of:
show, hide, toggle, dispose, enable, disable, toggleEnabled, update"""
return js.call_js('pop', self, behaviour)
Button.popover = popover
Link.popover = popover
Button.pop = pop
Link.pop = pop
You can then call the newly created popover
and pop
methods on Buttons and Links:
self.button = Button()
# Create a Button
self.button.popover(content='example text', title='Popover')
# Add a popover to your button when it is clicked.
self.button.popover(content='You hovered over the button', placement = 'bottom', trigger='hover')
# Add a popover to your button when you hover over it.
You can also add Forms to the popovers:
from .Form2 import Form2
# ...
self.button.popover(content=Form2())
Form2 will also be aware of the ‘popper’ as it inherits a self.popper
attribute.
To see the full source code, clone the app: