Anvil Components
The Anvil User Interface is made up of components. Even Forms are a special kind of component.
Some components display information to the user, such as Labels and Images. Others take user input, such as TextBoxes and Buttons.
You control the content, appearance and behaviour of your components by setting their Properties. You can set these properties while you’re building your app using the Properties Panel or the Object Palette. You can also set them while the app is running using Python code.
You can make components interactive using Events. These are Python functions that run when something happens - when the user clicks on the component, or enters text, or when the component appears on the screen for the first time.
Many components can contain other components - these are called ‘containers’. Containers can contain other containers, allowing nested structures. This is how Anvil User Interfaces get their structure.
Building UIs in Anvil consists of combining components, properties, events and containers to get the result you want. Let’s look at each concept in more detail.
Components
Components are the things you add to your Form to build your UI. These include Buttons, Labels, TextBoxes and similar widgets.
The entire list of Anvil components is given in this section of the docs. The categories are:
- Basic Components - things like Labels, Links and Images.
- Containers - these group components together and define where they sit on the page.
- RepeatingPanels - these repeat the same group of components for all items in a given list.
- Forms - these are special containers that appear in the App Browser and can and play the role of a ‘page’ in Anvil.
- Data Grids - these are an easy and powerful way to create a paginated table.
- Plots - these display data using Plotly.
- Maps - Google Maps in Python.
- Canvas - these provide area where you can draw graphics, like an HTML canvas but in Python.
When you design a Form in the Form editor, you place components on the Form by dragging and dropping them. Anvil stores that layout in the configuration for your app, and knows to create those components at runtime when that particular Form is displayed.
To delete a component, select it in the Form Editor and press the Delete or Backspace key on your keyboard.
Every component is available in Python as an attribute of the Form object. So when you add a Button to your Form in the designer and give it the name button_1
:
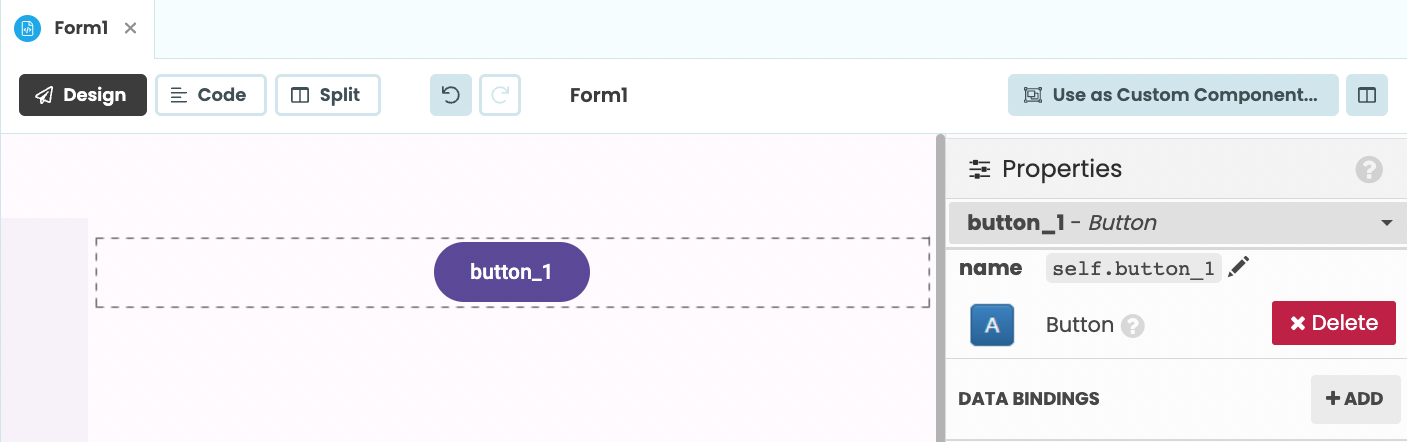
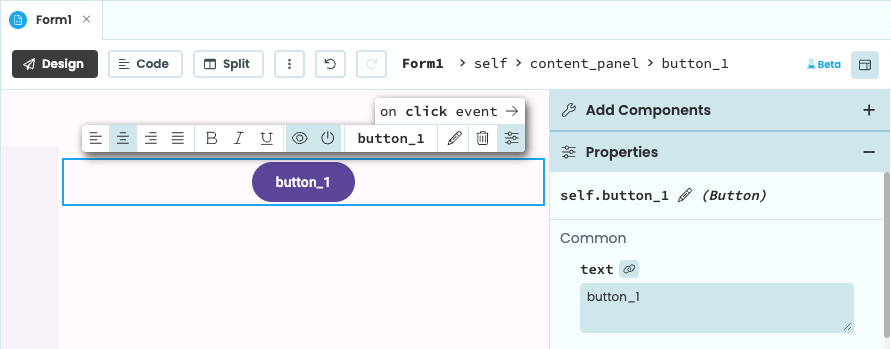
you can refer to it within your code as self.button_1
:
class Form1(Form1Template):
# .. Somewhere inside Form1 ...
self.button_1.text = "Click me"
However, this isn’t the only way to do it. Components are just Python classes, so you can construct new instances entirely in code:
class Form1(Form1Template):
# .. Somewhere inside Form1 ...
button_1 = Button()
This Button exists in Python, but it has not been added to the Form yet - we’ll show you how in the Containers section.
First we’ll cover more about what you can do with components - properties and events.
Properties
Components have properties that determine how they look and how they behave. Properties can be modified in the Anvil Editor using the Properties Panel (on the right below the Toolbox):
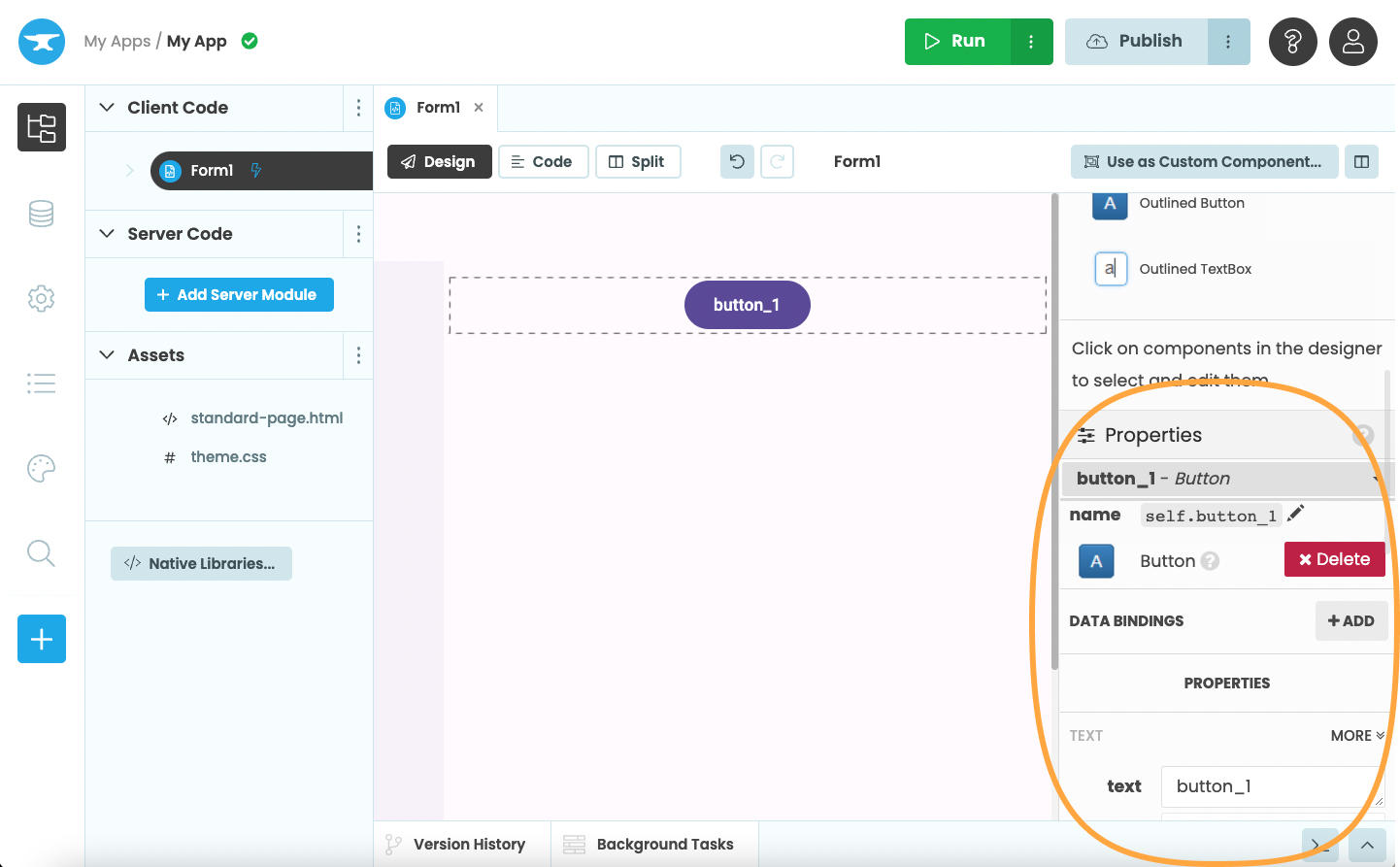
The Properties Panel allows you to edit a component’s content, appearance and behaviour.
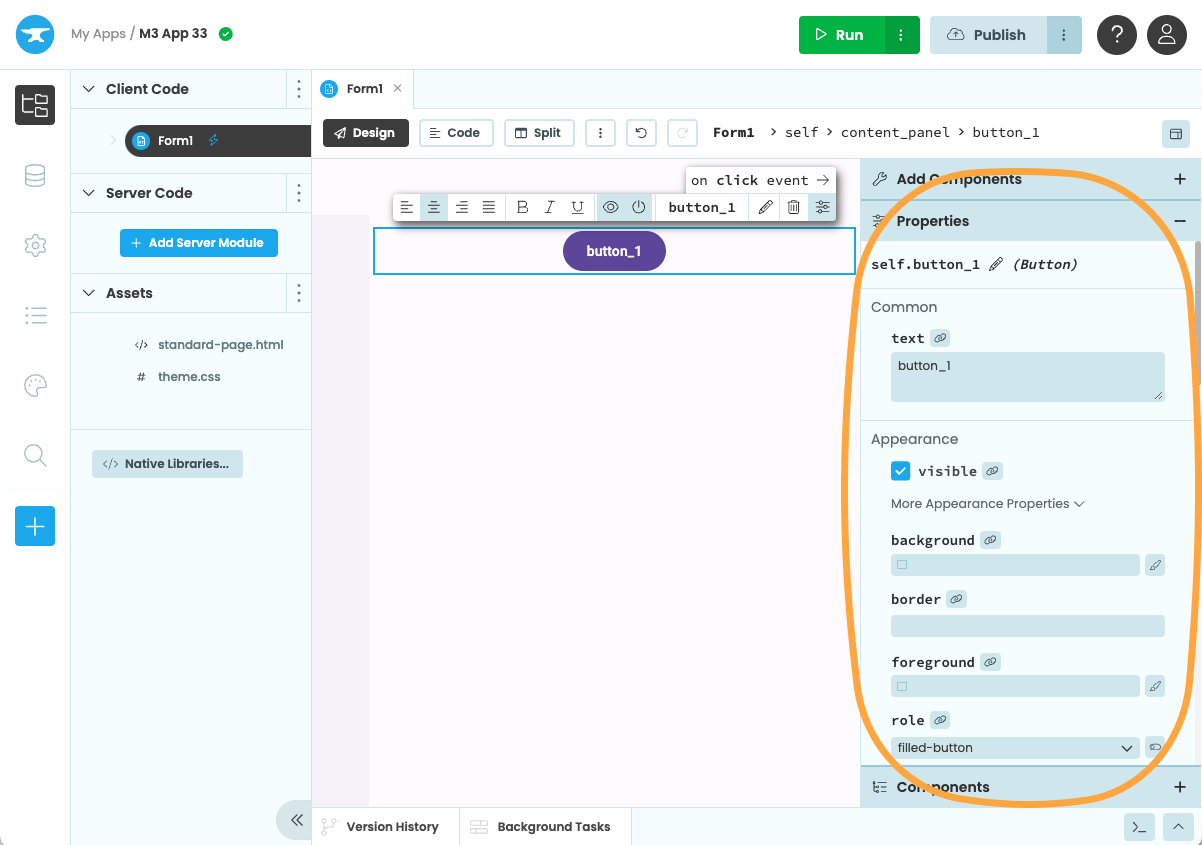
The Properties Panel allows you to edit a component’s content, appearance and behaviour.
You can also change some of the most common component properties from the Object Palette, which will show up above the component when you select it.
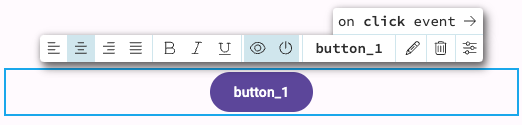
The Object Palette allows you to quickly modify
a component’s most common properties
You can adjust a component’s appearance by setting a property:
self.button_1.text = "Click me"
You can also read data from a component by reading properties (for example the text
property of a
TextBox component contains whatever text the user has entered in the box).
latest_user_input = self.text_box_1.text
The properties of all Anvil’s built-in components can be found in the API Reference.
If you’re creating components in code, you can pass in initial values for the properties when you create the component, for example Button(text="Click me")
.
Every component also has a property called tag
, which is for you to store any extra data you like. By default, it’s an empty object that you can store attributes on:
self.my_textbox.tag.foo = "bar"
Events
Components can also raise events. For example, when a Button is clicked, it raises the click
event.
You can see what events a component raises at the bottom of the Properites Panel. This example is for a Button component:
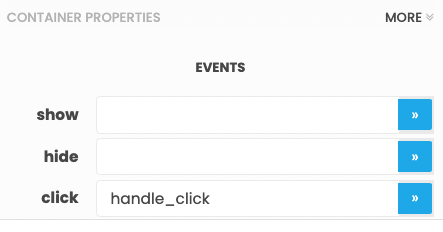
This Button calls self.handle_click
when it is clicked.
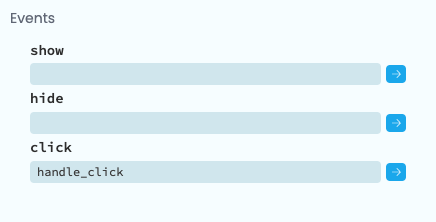
This Button calls self.handle_click
when it is clicked.
To define what to do when an event is raised, you can enter a method name in the box. This is called ‘binding’ a method to an event. In the example above, the click
event has the handle_click
method bound to it.
The methods you bind must belong to the Form that the component is on. If the Button is in Form1
and it has handle_click
bound to it, then your Form1
code must look like this:
class Form1(Form1Template):
# ...
def handle_click(self, **event_args):
# Any code you write here will run before the button is clicked
There’s a shortcut to create event handlers: you can click on the blue arrows next to the event to get a click handler automatically defined for you in code:
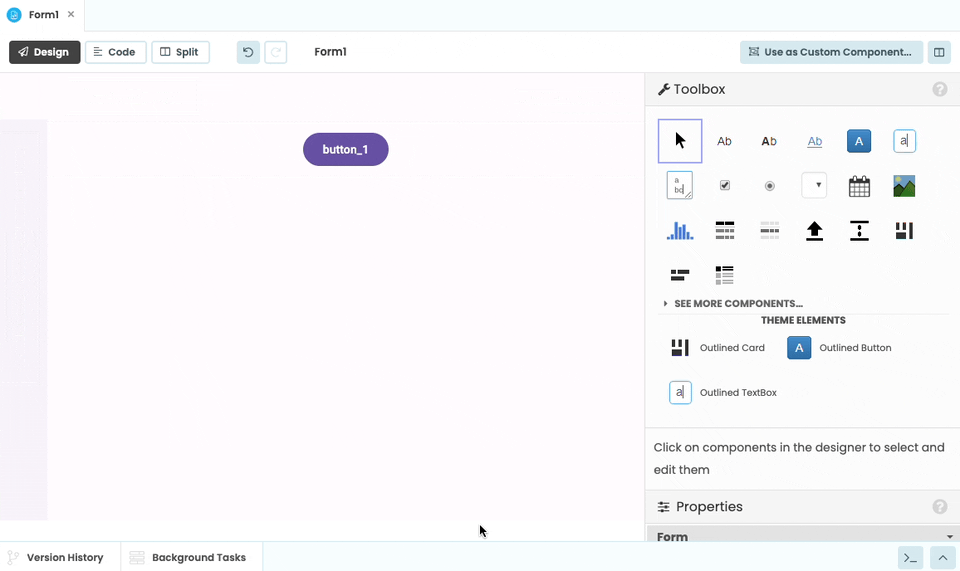
Automatically creating an event handler.
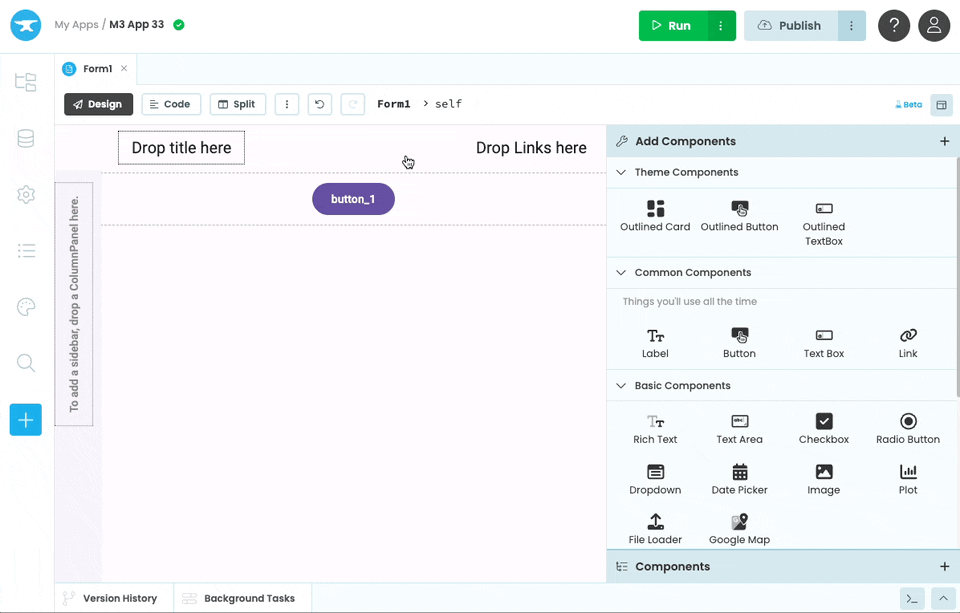
Automatically creating an event handler.
You can also set the most common event for each component from the Object Palette shortcut:
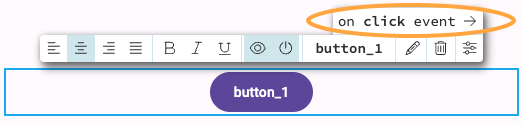
The Object Palette shorcut for the
Button component sets the click
event
For a full list of what events each component raises, see the API Reference.
For more detail on raising component events in Anvil, see our documentation on Events
Containers
Some components can contain other components. We call these containers, and they all inherit from the Container
class. (All Forms are also containers.)
For a full list of container components, see Components: Containers.
You can add a component to a container in Python by calling the container’s add_component()
method.
btn = Button()
self.linear_panel_1.add_component(btn)
You can call a container’s get_components()
method to get a list of all the components that have been added to it.
Parents and children
You can look up a component’s container with the .parent
attribute. If a component has not yet been added to a container, its .parent
attribute is None
.
print(btn.parent)
# prints "<anvil.XYPanel object>" for a button inside an XY Panel
Try to avoid using multiple .parent
attributes chained together to go up the component hierarchy.
You will probably find your code is more robust if you use get_open_form()
to get a reference to the
currently-open Form and find the component you’re looking for from there.
Removing components
To remove a component from a container, call the component’s remove_from_parent()
method.
btn.remove_from_parent()
To remove all components from a container, call the container’s clear()
method.
self.xy_panel_1.clear()
Raising an event on all children
To raise an event on all components in a container, call the raise_event_on_children
method
container.raise_event_on_children(event_name, extra_args)
This takes exactly the same parameters as component.raise_event
, and is subject to the same constraints on event names.
It is especially useful when you want to signal to all children of a RepeatingPanel without already having explicit references to those children.
Container Properties
Container Properties are properties that control the relationship between a component and the container it is in; the argument names and their meanings depend on the type of container. They are visible in the Properties Panel in the Anvil Editor. They can also be passed as keyword arguments to add_component
.
For example, components added to XYPanels have x
, y
, and width
properties, which determine the position of the component. You can specify them in the designer:
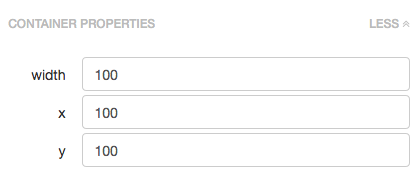
Container properties are at the bottom of the
Properties Panel
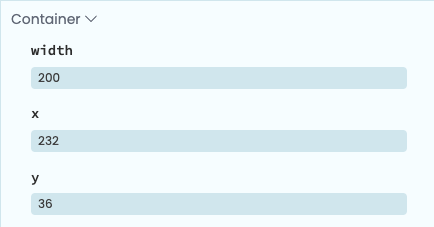
Container properties are at the bottom of the
Properties Panel
Or you can pass them as keyword arguments when you call add_component()
in your code:
btn = Button(text="Click me")
self.xy_panel_1.add_component(btn, x=100, y=100)
For more information on the container properties available for each container type, consult the Anvil API Reference.
Data Bindings
Read the full documentation on Data Bindings for more detail about Data Bindings and when you might find them useful.
Data Bindings are a tidy way to define a relationship between a component’s property and some underlying data - they associate a property of a component with a single Python expression. The relationship is defined in one place so you don’t need to re-write the code in many different parts of your app.
Data Bindings are defined in the Properties Panel of the Anvil Editor. You select a property from the Dropdown and write a Python expression in the box.
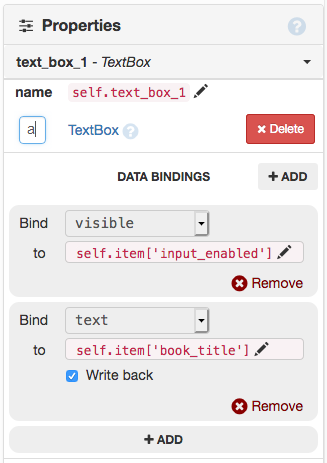
Properties Panel for a TextBox
with two Data Bindings.
Data Bindings are defined in the Properties Panel of the Anvil Editor. Click on the link icon next to the property that you wish to bind and write a Python expression in the box.
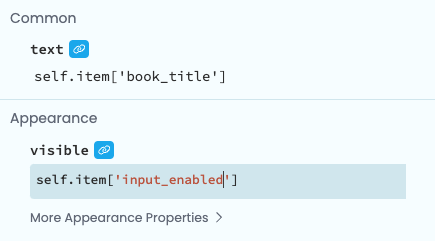
Properties Panel for a Button with
two Data Bindings.
self
here refers to the Form that this component is onThe Python expression can use any attribute or method of the Form that the component is on. The Form is available as self
- you
can use anything that looks like self.<something>
The Data Binding is evaluated when any of these things happen:
- when
self.init_components(**properties)
gets called in the__init__
method - when
self.refresh_data_bindings()
is called - when
self.item
is set
A component can have any number of Data Bindings (one for each of its properties).
Do you still have questions?
Our Community Forum is full of helpful information and Anvil experts.